3.2.1. The container
The org.springframework.beans.factory.BeanFactory is the actual representation of the Spring IoC container that is responsible for containing and otherwise managing the aforementioned beans.
The BeanFactory interface is the central IoC container interface in Spring. Its responsibilities include instantiating or sourcing application objects, configuring such objects, and assembling the dependencies between these objects.
There are a number of implementations of the BeanFactory interface that come supplied straight out-of-the-box with Spring. The most commonly used BeanFactory implementation is the XmlBeanFactory class. This implementation allows you to express the objects that compose your application, and the doubtless rich interdependencies between such objects, in terms of XML. The XmlBeanFactory takes this XML configuration metadata and uses it to create a fully configured system or application.
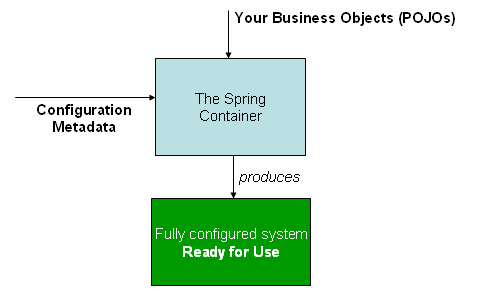
The Spring IoC container
As can be seen in the above image, the Spring IoC container consumes some form of configuration metadata; this configuration metadata is nothing more than how you (as an application developer) inform the Spring container as to how to “instantiate, configure, and assemble [the objects in your application]”. This configuration metadata is typically supplied in a simple and intuitive XML format. When using XML-based configuration metadata, you write bean definitions for those beans that you want the Spring IoC container to manage, and then let the container do it's stuff.
![]() | Note |
---|---|
XML-based metadata is by far the most commonly used form of configuration
metadata. It is not however the only form of configuration metadata that is allowed. The Spring IoC container itself is totally decoupled from the format in which this configuration metadata is actually written. At the time of writing, you can supply this configuration metadata using either XML, the Java properties format, or programmatically (using Spring's public API). The XML-based configuration metadata format really is simple though, and so the remainder of this chapter will use the XML format to convey key concepts and features of the Spring IoC container. |
Please be advised that in the vast majority of application scenarios, explicit user code is not required to instantiate one or more instances of a Spring IoC container. For example, in a web application scenario, a simple eight (or so) lines of absolutely boilerplate J2EE web descriptor XML in the web.xml file of the application will typically suffice (see Section 3.8.4, “Convenient ApplicationContext instantiation for web applications”).
Spring configuration consists of at least one bean definition that the container must manage, but typically there will be more than one bean definition. When using XML-based configuration metadata, these beans are configured as <bean/> elements inside a top-level <beans/> element.
These bean definitions correspond to the actual objects that make up your application. Typically you will have bean definitions for your service layer objects, your data access objects (DAOs), presentation objects such as Struts Action instances, infrastructure objects such as Hibernate SessionFactory instances, JMS Queuereferences, etc. (the possibilities are of course endless, and are limited only by the scope and complexity of your application). (Typically one does not configure fine-grained domain objects in the container.)
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.0.xsd"> <bean id="..." class="..."> <!-- collaborators and configuration for this bean go here --> </bean> <bean id="..." class="..."> <!-- collaborators and configuration for this bean go here --> </bean> <!-- more bean definitions go here... --> </beans>
Instantiating a Spring IoC container is easy; find below some examples of how to do just that:
Resource resource = new FileSystemResource("beans.xml"); BeanFactory factory = new XmlBeanFactory(resource);
... or...
ClassPathResource resource = new ClassPathResource("beans.xml"); BeanFactory factory = new XmlBeanFactory(resource);
... or...
ApplicationContext context = new ClassPathXmlApplicationContext(
new String[] {"applicationContext.xml", "applicationContext-part2.xml"});
// of course, an ApplicationContext is just a BeanFactory
BeanFactory factory = context;
It can often be useful to split up container definitions into multiple XML files. One way to then load an application context which is configured from all these XML fragments is to use the application context constructor which takes multiple Resource locations. With a bean factory, a bean definition reader can be used multiple times to read definitions from each file in turn.
Generally, the Spring team prefers the above approach, since it keeps container configuration files unaware of the fact that they are being combined with others. An alternate approach is to use one or more occurrences of the <import/> element to load bean definitions from another file (or files). Let's look at a sample:
<beans> <import resource="services.xml"/> <import resource="resources/messageSource.xml"/> <import resource="/resources/themeSource.xml"/> <bean id="bean1" class="..."/> <bean id="bean2" class="..."/> </beans>
In this example, external bean definitions are being loaded from 3 files, services.xml, messageSource.xml, and themeSource.xml. All location paths are considered relative to the definition file doing the importing, so services.xml in this case must be in the same directory or classpath location as the file doing the importing, while messageSource.xmland themeSource.xml must be in a resources location below the location of the importing file. As you can see, a leading slash is actually ignored, but given that these are considered relative paths, it is probably better form not to use the slash at all.
The contents of the files being imported must be fully valid XML bean definition files according to the Schema or DTD, including the top level <beans/> element.
As mentioned previously, a Spring IoC container manages one or more beans. These beans are created using the instructions defined in the configuration metadata that has been supplied to the container (typically in the form of XML <bean/> definitions).
Within the container itself, these bean definitions are represented as BeanDefinition objects, which contain (among other information) the following metadata:
- a package-qualified class name: this is normally the actual implementation class of the bean being defined. However, if the bean is to be instantiated by invoking a staticfactory method instead of using a normal constructor, this will actually be the class name of the factory class.
- bean behavioral configuration elements, which state how the bean should behave in the container (prototype or singleton, autowiring mode, initialization and destruction callbacks, and so forth).
- constructor arguments and property values to set in the newly created bean. An example would be the number of connections to use in a bean that manages a connection pool (either specified as a property or as a constructor argument), or the pool size limit.
- other beans which are needed for the bean to do its work, that is collaborators (also called dependencies).
The concepts listed above directly translate to a set of properties that each bean definition consists of. Some of these properties are listed below, along with a link to further documentation about each of them.
Table 3.1. The bean definition
Feature
|
Explained
in...
|
class
|
|
name
|
|
scope
|
|
constructor
arguments
|
|
properties
|
|
autowiring
mode
|
|
dependency
checking mode
|
|
lazy-initialization
mode
|
|
initialization
method
|
|
destruction
method
|
Besides bean definitions which contain information on how to create a specific bean, certain BeanFactory implementations also permit the registration of existing objects that have been created outside the factory (by user code). The DefaultListableBeanFactory class supports this through the registerSingleton(..) method. Typical applications solely work with beans defined through metadata bean definitions, though.
Every bean has one or more ids (also called identifiers, or names; these terms refer to the same thing). These ids must be unique within the container the bean is hosted in. A bean will almost always have only one id, but if a bean has more than one id, the extra ones can essentially be considered aliases.
When using XML-based configuration metadata, you use the 'id' or 'name' attributes to specify the bean identifier(s). The 'id' attribute allows you to specify exactly one id, and as it is a real XML element ID attribute, the XML parser is able to do some extra validation when other elements reference the id; as such, it is the preferred way to specify a bean id. However, the XML specification does limit the characters which are legal in XML IDs. This is usually not a constraint, but if you have a need to use one of these special XML characters, or want to introduce other aliases to the bean, you may also or instead specify one or more bean ids, separated by a comma (,), semicolon (;), or whitespace in the 'name' attribute.
Please note that you are not required to supply a name for a bean. If no name is supplied explicitly, the container will generate a (unique) name for that bean. The motivations for not supplying a name for a bean will be discussed later (one use case is inner beans).
In a bean definition itself, you may supply more than one name for the bean, by using a combination of up to one name specified via the id attribute, and any number of other names via the name attribute. All these names can be considered equivalent aliases to the same bean, and are useful for some situations, such as allowing each component used in an application to refer to a common dependency using a bean name that is specific to that component itself.
Having to specify all aliases when the bean is actually defined is not always adequate however. It is sometimes desirable to introduce an alias for a bean which is defined elsewhere. In XML-based configuration metadata this may be accomplished via the use of the standalone <alias/> element. For example:
<alias name="fromName" alias="toName"/>
In this case, a bean in the same container which is named 'fromName', may also after the use of this alias definition, be referred to as 'toName'.
As a concrete example, consider the case where component A defines a DataSource bean called componentA-dataSource, in its XML fragment. Component B would however like to refer to the DataSource as componentB-dataSource in its XML fragment. And the main application, MyApp, defines its own XML fragment and assembles the final application context from all three fragments, and would like to refer to the DataSource as myApp-dataSource. This scenario can be easily handled by adding to the MyApp XML fragment the following standalone aliases:
<alias name="componentA-dataSource" alias="componentB-dataSource"/> <alias name="componentA-dataSource" alias="myApp-dataSource" />
Now each component and the main app can refer to the dataSource via a name that is unique and guaranteed not to clash with any other definition (effectively there is a namespace), yet they refer to the same bean.
A bean definition can be seen as a recipe for creating one or more actual objects. The container looks at the recipe for a named bean when asked, and uses the configuration metadata encapsulated by that bean definition to create (or acquire) an actual object.
If you are using XML-based configuration metadata, you can specify the type (or class) of object that is to be instantiated using the 'class' attribute of the <bean/> element. This 'class' attribute (which internally eventually boils down to being a Class property on a BeanDefinition instance) is normally mandatory (see Section 3.2.3.2.3, “Instantiation using an instance factory method” and Section 3.6, “Bean definition inheritance” for the two exceptions) and is used for one of two purposes. The class property specifies the class of the bean to be constructed in the much more common case where the container itself directly creates the bean by calling its constructor reflectively (somewhat equivalent to Java code using the 'new' operator). In the less common case where the container invokes a static, factory method on a class to create the bean, the class property specifies the actual class containing the static factory method that is to be invoked to create the object (the type of the object returned from the invocation of the static factory method may be the same class or another class entirely, it doesn't matter).
When creating a bean using the constructor approach, all normal classes are usable by and compatible with Spring. That is, the class being created does not need to implement any specific interfaces or be coded in a specific fashion. Just specifying the bean class should be enough. However, depending on what type of IoC you are going to use for that specific bean, you may need a default (empty) constructor.
Additionally, the Spring IoC container isn't limited to just managing true JavaBeans, it is also able to manage virtually any class you want it to manage. Most people using Spring prefer to have actual JavaBeans (having just a default (no-argument) constructor and appropriate setters and getters modeled after the properties) in the container, but it is also possible to have more exotic non-bean-style classes in your container. If, for example, you need to use a legacy connection pool that absolutely does not adhere to the JavaBean specification, Spring can manage it as well.
When using XML-based configuration metadata you can specify your bean class like so:
<bean id="exampleBean" class="examples.ExampleBean"/> <bean name="anotherExample" class="examples.ExampleBeanTwo"/>
The mechanism for supplying arguments to the constructor (if required), or setting properties of the object instance after it has been constructed, will be described shortly.
When defining a bean which is to be created using a static factory method, along with the class attribute which specifies the class containing the static factory method, another attribute named factory-method is needed to specify the name of the factory method itself. Spring expects to be able to call this method (with an optional list of arguments as described later) and get back a live object, which from that point on is treated as if it had been created normally via a constructor. One use for such a bean definition is to call staticfactories in legacy code.
The following example shows a bean definition which specifies that the bean is to be created by calling a factory-method. Note that the definition does not specify the type (class) of the returned object, only the class containing the factory method. In this example, the createInstance() method must be a static method.
<bean id="exampleBean" class="examples.ExampleBean2" factory-method="createInstance"/>
The mechanism for supplying (optional) arguments to the factory method, or setting properties of the object instance after it has been returned from the factory, will be described shortly.
In a fashion similar to instantiation via a static factory method, instantiation using an instance factory method is where the factory method of an existing bean from the container is invoked to create the new bean.
To use this mechanism, the 'class' attribute must be left empty, and the 'factory-bean' attribute must specify the name of a bean in the current (or parent/ancestor) container that contains the factory method. The factory method itself must still be set via the 'factory-method' attribute (as seen in the example below).
<!-- the factory bean, which contains a method called createInstance() --> <bean id="myFactoryBean" class="..."> ... </bean> <!-- the bean to be created via the factory bean --> <bean id="exampleBean" factory-bean="myFactoryBean" factory-method="createInstance"/>
Although the mechanisms for setting bean properties are still to be discussed, one implication of this approach is that the factory bean itself can be managed and configured via DI.
A BeanFactory is essentially nothing more than the interface for an advanced factory capable of maintaining a registry of different beans and their dependencies. The BeanFactoryenables you to read bean definitions and access them using the bean factory. When using just the BeanFactory you would create one and read in some bean definitions in the XML format as follows:
InputStream is = new FileInputStream("beans.xml"); BeanFactory factory = new XmlBeanFactory(is);
Basically that's all there is to it. Using getBean(String) you can retrieve instances of your beans; the client-side view of the BeanFactory is surprisingly simple. TheBeanFactory interface has only six methods for client code to call:
- boolean containsBean(String): returns true if the BeanFactory contains a bean definition or bean instance that matches the given name
- Object getBean(String): returns an instance of the bean registered under the given name. Depending on how the bean was configured by the BeanFactory configuration, either a singleton and thus shared instance or a newly created bean will be returned. A BeansException will be thrown when either the bean could not be found (in which case it'll be a NoSuchBeanDefinitionException), or an exception occurred while instantiating and preparing the bean
- Object getBean(String, Class): returns a bean, registered under the given name. The bean returned will be cast to the given Class. If the bean could not be cast, corresponding exceptions will be thrown (BeanNotOfRequiredTypeException). Furthermore, all rules of the getBean(String) method apply (see above)
- Class getType(String name): returns the Class of the bean with the given name. If no bean corresponding to the given name could be found, aNoSuchBeanDefinitionException will be thrown
- boolean isSingleton(String): determines whether or not the bean definition or bean instance registered under the given name is a singleton (bean scopes such as singleton are explained later). If no bean corresponding to the given name could be found, a NoSuchBeanDefinitionException will be thrown
- String[] getAliases(String): Return the aliases for the given bean name, if any were defined in the bean definition
No comments:
Post a Comment